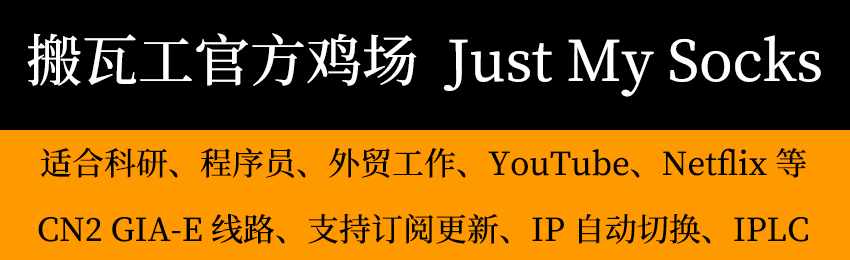
打开凤凰新闻,查看更多高清图片
在 Java 中,如何用Swing实现打字游戏.需要掌握多线程,JFrame的创建方法。多线程的创建方法,一个是继承Thread类,一个是实现Runnable接口。框架的实现,直接创建一个类继承JFrame.
基本思路是,1.创建一个JFrame 2.启动十个线程,每个线程携带了一个JLabel,用到了随机函数,每个JLabel的刚出现的时候,他们的y坐标都是一样的,x坐标是随机的(随机范围在JFrame的宽度内.JLabel显示的值,是Ascii对应的拉丁字母 .3.线程控制每个JLael的y坐标增加,这样字母就在往下掉 4.响应键盘事件
//载入包
import java.awt.*;
import javax.swing.*;
import java.util.*;
import java.awt.event.*;
import java.applet.*;
// 创建一个线程
class MyThread extends Thread {
int height=0;
// JLabel的x坐标
int intx=0;
// JLabel 的y坐标
int inty=0;
static int com=0;
JLabel lb1;
public MyThread(ThreadGroup g,String name,int width,int fheight){
this.setName(name);
height=fheight;
// 设置JLabel显示的大写字母,通过随机值控制.65是大写A的Ascii值
lb1=new JLabel(""+(char)(int)(Math.random()*26+65));
// 设置JLabel的背景颜色,红,绿,蓝组成自然界所有色彩
lb1.setForeground(new Color((int)(Math.random()*250),(int)(Math.random()*250),(int)(Math.random()*250)));
intx=(int)(Math.random()*(width-20));
//线程启动
start();
}
public void run(){
try{
while(true){
//睡眠50毫秒往下掉
sleep((int)(Math.random()*50));
lb1.setBounds(intx,inty,15,15);
//改变y坐标
inty++;
if(inty>=(height-20)){
lb1.show(false);
MyThread.com++;
break;
}
}
}catch(Exception e){}
}
}
//创建窗口
class TestKey extends JFrame
{
JButton b1,b2;
static int index=0;
Container conent=getContentPane();
static int idd=0;
static int count=0;
int ixx;
static ThreadGroup dg = new ThreadGroup("group1");
static ThreadGroup dg1;
static MyThread t1;
Thread list[];
public TestKey() throws ArrayStoreException
{
setSize(400,600);
b1=new JButton("开始");
b2=new JButton("结束");
conent.setLayout(null);
b1.setBounds(getWidth()/2-100,getHeight()-70,80,30);
b2.setBounds(getWidth()/2+50,getHeight()-70,80,30);
conent.add(b1);
conent.add(b2);
conent.setBackground(Color.blue);
Container cont=getContentPane();
conent.setBackground(Color.white);
show();
//响应按钮事件
b1.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent even)
{
for(int i=0;i<10;i++)
{
t1 =new MyThread(dg,"t"+i,getWidth(),getHeight());
getContentPane().add(t1.lb1);
}
}
});
b2.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent even)
{
int num=conent.getComponentCount()-2;
for(int i=2;i
{
conent.remove(2);
conent.repaint();
}
if((float)num/count<=0.02)
JOptionPane.showMessageDialog(null,"共有"+count+"个字母\n"+"只有"+num+"个未打掉!\n您真是高手,佩服,佩服!");
else if((float)num/count<=0.15)
JOptionPane.showMessageDialog(null,"共有"+count+"个字母\n"+"有"+num+"个未打掉!\n水平不错,继续努力吧!");
else if((float)num/count<=0.3)
JOptionPane.showMessageDialog(null,"共有"+count+"个字母\n"+"有"+num+"个未打掉!\n您很有潜力,不要虚度光阴哦!");
else if((float)num/count<=0.4)
JOptionPane.showMessageDialog(null,"共有"+count+"个字母\n"+"有"+num+"个未打掉!\n挺危险的,加把劲啊!");
else
JOptionPane.showMessageDialog(null,"共有"+count+"个字母\n"+"有"+num+"个未打掉!\n不蛮你,你真的很差劲,抓紧练习吧!");
}
});
//监听窗口事件
addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent event)
{
if(conent.getComponentCount()==2)
JOptionPane.showMessageDialog(null,"您真的要退出吗?\n记得经常来练习啊!");
else
JOptionPane.showMessageDialog(null,"您确定要退出吗?\n我还没为您评分的啊!");
System.exit(0);
}
});
b1.addKeyListener(new KeyAdapter()
{
public void keyTyped(KeyEvent event)
{
list = new Thread[dg.getParent().activeCount()];
int count = dg.getParent().enumerate(list);
for(int i=0;i
{
try
{
MyThread mt=null;
mt = (MyThread)(list[i]);// instanceof MyThread)
if(event.getKeyChar()== mt.lb1.getText().charAt(0))
{
mt.lb1.setVisible(false);
MyThread hh=new MyThread(dg,"t"+i,getWidth(),getHeight());
getContentPane().add(hh.lb1);
}
}catch(Exception e){}
}
}
});
}
public static void main(String args[]){
TestKey mf=new TestKey();
Container cont=mf.getContentPane();
cont.setBackground(Color.white);
cont.setLayout(null);
//创建十个线程,携带十个字母往下掉
for(int i=0;i<10;i++)
{
t1 =new MyThread(dg,"t"+i,mf.getWidth(),mf.getHeight());
cont.add(t1.lb1);
}
}
}
大家有不同的实现方式,欢迎在下面留言