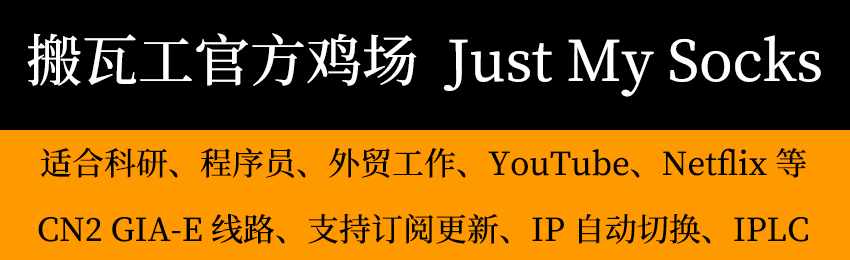
项目结构:
项目结构图
1:npm方式安装axios。
2:安装好后如项目结构图第二步,新建api文件夹,然后建config.js文件,在该文件中import axios.js。
import axios from "axios"==import axios from "axios.js";因为vscode会自动检测.js和.vue后缀文件。
config.js代码:
import axios from "axios"; // 创建一个axios实例 const service = axios.create({ // 请求超时时间 timeout: 3000 }); // 添加请求拦截器 // service.interceptors.request.use( // config => { // //console.log(config); // return config; // }, // err => { // console.log(err); // } // ); //响应拦截器。 // service.interceptors.response.use( // response => { // let res = {}; // //console.log("响应拦截器。" + res); // res.status = response.status; // res.data = response.data; // return res; // }, // err => console.log(err) // ); export default service;3:在main.js中引入第二步中的config.js文件(import http from "@/api/config"),然后挂载到Vue原型上(Vue.prototype.$http = http; //挂载到vue原型上)。
代码:
import Vue from "vue"; import App from "./App.vue"; import router from "./router"; //在根目录里找不到router.js的话,会默认去router目录下找对应的路由js文件。2019.12.15 import store from "./store"; // 全局配置 import "@/assets/scss/reset.scss"; import "element-ui/lib/theme-chalk/index.css"; import http from "@/api/config"; import "./mock"; // 第三方包 import ElementUI from "element-ui"; //import JsonExcel from "vue-json-excel"; //Vue.component("downloadExcel", JsonExcel); Vue.use(ElementUI); Vue.prototype.$http = http; //挂载到vue原型上。 Vue.prototype.$baseUrl = ":5001"; Vue.config.productionTip = false; new Vue({ router, store, render: h => h(App) }).$mount("#app");4:使用挂载好的axios获取数据:
<template> <div style="padding: 20px"> <div class="main"> <el-form :model="form" label-width="120"> <el-form-item label="用户名"> <el-input v-model="form.username" clearable></el-input> </el-form-item> <el-form-item label="密码"> <el-input v-model="form.password" type="password" show-password></el-input> </el-form-item> <el-form-item align="right"> <el-button type="primary" @click="login">登录</el-button> </el-form-item> </el-form> </div> <!-- <img :src="backgrdImg" /> --> <div class="container"> <div class="battery"></div> </div> </div> </template> <script> export default { data() { return { form: { username: "", password: "" }, backgrdImg: require("../../assets/images/bg.jpg") }; }, methods: { login() { this.$http.post("api/permission/getMenu", this.form).then(res => { res = res.data; if (res.code === 20000) { this.$store.commit("clearMenu"); this.$store.commit("setMenu", res.data.menu); this.$store.commit("setToken", res.data.token); this.$store.commit("addMenu", this.$router); this.$router.push({ name: "home" }); } else { this.$message.warning(res.data.message); } }); //console.log("111" + this.$baseUrl); this.$http.get(this.$baseUrl + "/weatherforecast").then(res => { console.log(res.data); res = res.data; }); //请求接口参数类型为[formbody],且参数为类。Account对应为类中属性。2019.12.30 this.$http.post(this.$baseUrl + "/weatherforecast", { Account: "longdb", Pw: "123" }).then(res => { console.log(res.data); res = res.data; }); }, mounted() { console.log("333" + this.$route.path); } } }; </script> <style lang="scss" scoped> .el-form { width: 100%; margin: auto; padding: 10px; height: 300px; background-color: rgb(23, 156, 156); border-radius: 5%; } .main { position: absolute; z-index: 10; right: 10%; top: 30%; } img { width: 100%; height: 100%; position: relative; z-index: 8; } .container { position: relative; width: 100%; height: 100%; margin: auto; // z-index: 9; } .battery { // height: 220px; height: 550px; box-sizing: border-box; border-radius: 15px 15px 5px 5px; filter: drop-shadow(0 1px 3px rgba(0, 0, 0, 0.22)); background: #fff; z-index: 9; // &::before { // content: "longdb"; // position: absolute; // width: 26px; // height: 10px; // left: 50%; // top: 0; // transform: translate(-50%, -10px); // border-radius: 5px 5px 0 0; // background: rgba(240, 240, 240, 0.88); // } &::after { content: ""; position: absolute; left: 0; right: 0; bottom: 0; top: 90%; background: linear-gradient(to bottom, #7abcff 0%, #00bcd4 44%, #2196f3 100%); border-radius: 0px 0px 5px 5px; box-shadow: 0 14px 28px rgba(33, 150, 243, 0), 0 10px 10px rgba(9, 188, 215, 0.08); animation: charging 10s linear infinite; filter: hue-rotate(90deg); } } @keyframes charging { 50% { box-shadow: 0 14px 28px rgba(0, 150, 136, 0.83), 0px 4px 10px rgba(9, 188, 215, 0.4); } 95% { top: 5%; filter: hue-rotate(0deg); border-radius: 0 0 5px 5px; box-shadow: 0 14px 28px rgba(4, 188, 213, 0.2), 0 10px 10px rgba(9, 188, 215, 0.08); } 100% { top: 0%; filter: hue-rotate(0deg); border-radius: 15px 15px 5px 5px; box-shadow: 0 14px 28px rgba(4, 188, 213, 0), 0 10px 10px rgba(9, 188, 215, 0.4); } } </style>