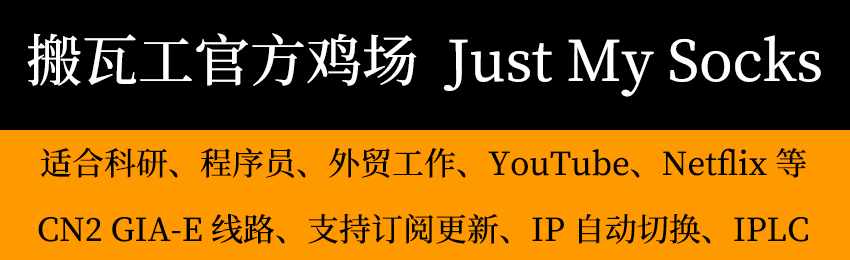
前言
文的文字及图片来源于网络,仅供学习、交流使用,不具有任何商业用途,版权归原作者所有,如有问题请及时联系我们以作处理。
作者: 南小小川/南川笔记
PS:如有需要Python学习资料的小伙伴可以加点击下方链接自行获取
?id=3054cce4add8a909e784ad934f956cef
本教程完全基于Python3版本,主要使用Chrome浏览器调试网页、Scrapy框架爬取数据、MongoDB数据库存储数据,选择这个组合的理由是成熟、稳定、快速、通行,此外可能会涉及Requests+BeautifulSoup解析、Redis数据库、Djiango/Flask框架等,适合已有一定爬虫基础的朋友学习爬取主流网站数据。
工作流程
根据前期查询、分析、总结,得到一条实现本项目的路径:
反爬分析
UA访问网易云只需要User-Agent是正常的即可,直接通过F12把自己的浏览器UI存入程序中。
IP网易云对于爬取过快的单击将会拉黑IP,根据使用校园网被拉黑的经历来看,网易云封IP的时间还是挺长的,可能接近1天,比新浪微博返回418要残忍很多,所以千万不要用校园网爬取网易云,不然被ban了你连正常的网易云都访问不了了。我自己使用的是芝麻代理,已经写成了DOWNLOAD_MIDDLEWARE,结合MongoDB数据库Scrapy在爬取中会自动切换、重新获得可用的代理IP。网上也有很多免费代理IP的网站,比如西刺等,Github上也有现成开源的动态爬取免费IP的项目,有些有点问题,但因为工程量的问题,我一直没用。
iFrame网易云的所有歌曲信息、评论等等,都是嵌在iFrame框架里的,这个要特别特别注意。具体的表现为,当你在程序中使用Requests或者Scrapy访问李荣浩的热门歌曲页面:时,你会得不到任何你想要的歌曲信息,但你把这个#号去掉,就可以得到了。但是当你用正常浏览器访问这两个网址时,都会跳转到第一个,因为浏览器对其进行了JavaScript渲染。这点非常重要,具体的直观测试方法,就是在浏览器页面内右键,可以看到有两个选项,一个是查看网页源代码(View Page Source),一个是查看框架源代码(View Frame Source),自己点点看就能明显地知道区别了。如果你是用Selenium等自动化程序访问的,不要忘了切换Frame才能得到自己想要的数据。
API网易云的很多数据其实是有API的,只是不去研究不知道,或者说没有公开开放,但你在知乎、简书、Github上能找到一些,本次项目里面的爬取评论部分就是用的知乎里面一位用户给出的VIP的API,帮了我非常大的忙,因为如果不是有这个VIP的API,我们就要走前端JavaScript解密,去破解网易云的Aes和RSA加密过程,这个代价就巨高了,而且爬取速度也绝非直接用API能比的。用代理IP爬取网易云主站信息大概每1000页就要死一个,但是爬评论的API,每十万页死一个差不多了,甚至也许都不会死(我的IP都是短期生存5-25分钟的,所以可能是自己死掉了)。
核心代码
以下是Scrapy中从歌手分类页到歌手专辑页再到专辑内的单曲页爬取链:
def start_requests(self): for area in self._seq_area: for kind in self._seq_kind: for initial in self._seq_cat_initial: cat = f{area}00{kind} artists_url = self.settings[HOST_ARTISTS].format(cat=cat, initial=initial) yield Request(artists_url, callback=self.parse_artists) def parse_artists(self, response): for singer_node in response.css(#m-artist-box li): response.meta[item] = singer_item = SingerItem() singer_item[_id] = singer_item[singer_id] = singer_id = \ int(singer_node.css(a.nm::attr(href)).re_first(\d+)) singer_item[crawl_time] = datetime.now() singer_item[singer_name] = singer_node.css(a.nm::text).get() singer_item[singer_desc_url] = self.get_singer_desc(singer_id) singer_item[singer_hot_songs] = response.urljoin(singer_node.css(a.nm::attr(href)).re_first(\S+)) singer_item[cat_name] = response.css(.z-slt::text).get() singer_item[cat_id] = int(response.css(.z-slt::attr(href)).re_first(\d+)) singer_item[cat_url] = response.urljoin(response.css(.z-slt::attr(href)).re_first(\S+)) yield singer_item yield Request(self.get_singer_albums(singer_id), callback=self.parse_albums) def parse_albums(self, response): for li in response.css(#m-song-module li): yield response.follow(li.css(a.msk::attr(href)).get(), callback=self.parse_songs) next_page = response.css(div.u-page a.znxt::attr(href)).get() if next_page: yield response.follow(next_page, callback=self.parse_albums) def parse_songs(self, response): album_item = AlbumItem() album_item[_id] = album_item[album_id] = int(re.search(id=(\d+), response.url).group(1)) album_item[album_name] = response.css(h2::text).get() album_item[album_author] = response.css(a.u-btni::attr(data-res-author)).get() album_item[album_author_id] = int(response.css(p.intr:nth-child(2) a::attr(href)).re_first(\d+)) album_item[album_authors] =[{name: a.css(::text).get(), href: a.css(::attr(href)).get()} for a in response.css(p.intr:nth-child(2) a)] album_item[album_time] = response.css(p.intr:nth-child(3)::text).get() album_item[album_url] = response.url album_item[album_img] = response.css(.cover img::attr(src)).get() album_item[album_company] = response.css(p.intr:nth-child(4)::text).re_first(\w+) album_item[album_desc] = response.xpath(string(//div[@id="album-desc-more"])).get() if \ response.css(#album-desc-more) else response.xpath(string(.//div[@class="n-albdesc"]/p)).get() # 用这个 span#cnt_comment_count::text 有些没有评论的会出问题,会变成“评论” album_item[album_comments_cnt] = int(response.css(#comment-box::attr(data-count)).get()) album_item[album_songs] = response.css(#song-list-pre-cache li a::text).getall() album_item[album_appid] = int(json.loads(response.css(script[type="application/ld+json"]::text).get())[appid]) yield album_item for li in response.css(#song-list-pre-cache li): song_item = SongItem() song_item[crawl_time] = datetime.now() song_item[song_name] = li.css(a::text).get() song_item[_id] = song_item[song_id] = int(li.css(a::attr(href)).re_first(\d+)) song_item[song_url] = response.urljoin(li.css(a::attr(href)).re_first(\S+)) yield song_item try: # 热歌信息在节点下,可以通过div#hotsong-list li a 得到歌曲的Id, href, name # 但是,可以通过下面的textarea节点得到更为详细的data,这个不能通过正则匹配[],不然会被一些歌曲名给套住 # 有些歌手没有热门歌曲,比如: #/artist?id=,当接近200万首歌的数据爬取完毕之后,我们启动评论爬虫,主要工作就是遍历数据库中还没有更新“评论数”这个字段的歌曲id,然后访问对应的评论api,得到我们想要的评论数据。
核心代码如下:
def start_requests(self): cursor = self.coll_song.find({comments_cnt: {$exists: False}}, no_cursor_timeout=True) for song_item in cursor: if self.settings.get(PARSE_ALL_COMMENTS): limit, offset = 100, 0 elif self.settings.get(PARSE_HOT_COMMENTS): limit, offset = 0, 0 else: limit, offset = 0, 1 comment_url = self.get_comment_page_url(song_item[song_id], limit=limit, offset=offset) yield Request(comment_url, dont_filter=False, callback=self.parse, meta={song_item: song_item, limit: limit, offset: offset}) cursor.close() def parse(self, response): json_data = json.loads(response.text) comment_item = CommentItem() comment_item[comment_url] = response.url.split(?)[0] comment_item[crawl_time] = datetime.now() comment_item[isMusician] = json_data[isMusician] comment_item[comments_cnt] = comments_cnt = json_data[total] comment_item[song_name] = response.meta[song_item][song_name] comment_item[singer_name] = response.meta[song_item][singer_name] comment_item[song_id] = song_id = response.meta[song_item][song_id] for comment_info in json_data.get(comments): comment_item.update(comment_info) comment_item[_id] = comment_info[commentId] yield comment_item for comment_info in json_data.get(hotComments): comment_item.update(comment_info) comment_item[_id] = comment_info[commentId] yield comment_item if self.settings.get("PARSE_ALL_COMMENTS") and json_data[more]: response.meta[offset] = new_offset = response.meta[offset] + 10 yield Request(self.get_comment_page_url(song_id, offset=new_offset), callback=self.parse, dont_filter=False, meta=response.meta) else: song_item = SongItem() song_item[_id] = response.meta[song_item][song_id] song_item[comments_cnt] = comments_cnt yield song_item小结
本项目提供了一个爬取网易云音乐的可行路径,即歌手分类 → 歌手 → 歌手的专辑 → 专辑内的单曲 → 单曲的评论,是一个非常广度的路径,如果全程爬完能得到3万歌手、20万专辑、200万首单曲的必要信息,可根据这些信息做歌手、专辑、单曲排序,制作歌单、热点追踪等等,很有意义。