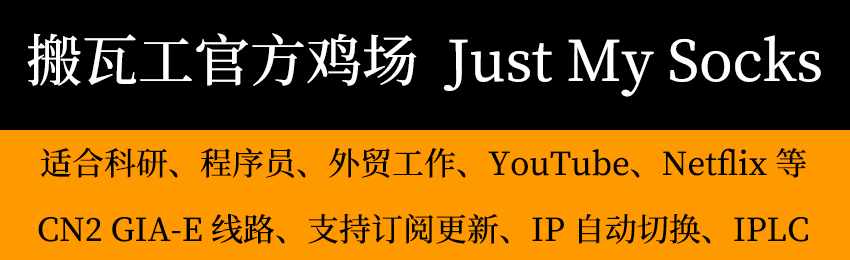
由于同源策略的限制,满足同源的脚本才可以获取资源。虽然这样有助于保障网络安全,但另一方面也限制了资源的使用。那么如何实现跨域呢,以下是实现跨域的一些方法。
目录
一、jsonp跨域
原理:script标签引入js文件不受跨域影响。不仅如此,带src属性的标签都不受同源策略的影响。
正是基于这个特性,我们通过script标签的src属性加载资源,数据放在src属性指向的服务器上,使用json格式。
由于我们无法判断script的src的加载状态,并不知道数据有没有获取完成,所以事先会定义好处理函数。服务端会在数据开头加上这个函数名,等全部加载完毕,便会调用我们事先定义好的函数,这时函数的实参传入的就是后端返回的数据了。
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros…
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>function callback(data) {alert(data.test);}</script><script src="./jsonp.js"></script></body></html>复制代码jsonp.js
callback({"test": 0});复制代码访问index.html弹窗便会显示0
该方案的缺点是:只能实现get一种请求。
二、document.domain + iframe跨域
此方案仅限主域相同,子域不同的应用场景。
比如百度的主网页是www.baidu.com,zhidao.baidu.com、news.baidu.com等网站是www.baidu.com这个主域下的子域。
实现原理:两个页面都通过js设置document.domain为基础主域,就实现了同域,就可以互相操作资源了。示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros…
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><iframe src=""></iframe><script>document.domain = mfaying.github.io;var t = 0;</script></body></html>复制代码child.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>document.domain = mfaying.github.io;alert(window.parent.t);</script></body></html>复制代码三、location.hash + iframe跨域
父页面改变iframe的src属性,location.hash的值改变,不会刷新页面(还是同一个页面),在子页面可以通过window.localtion.hash获取值。
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros…
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><iframe src="child.html#" style="display: none;"></iframe><script>var oIf = document.getElementsByTagName(iframe)[0];document.addEventListener(click, function () {oIf.src += 0;}, false);</script></body></html>复制代码child.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>window.onhashchange = function() {alert(window.location.hash.slice(1));}</script></body></html>复制代码点击index.html页面弹窗便会显示0
四、window.name + iframe跨域
原理:window.name属性在不同的页面(甚至不同域名)加载后依旧存在,name可赋较长的值(2MB)。
在iframe非同源的页面下设置了window的name属性,再将iframe指向同源的页面,此时window的name属性值不变,从而实现了跨域获取数据。示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros…
./parent/index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>var oIf = document.createElement(iframe);oIf.src = ;var state = 0;oIf.onload = function () {if (state === 0) {state = 1;oIf.src = ;} else {alert(JSON.parse(oIf.contentWindow.name).test);}}document.body.appendChild(oIf);</script></body></html>复制代码./parent/proxy.html 空文件,仅做代理页面
复制代码./child.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>window.name = {"test": 0}</script></body></html>复制代码五、postMessage跨域
postMessage是HTML5提出的,可以实现跨文档消息传输。
用法 getMessageHTML.postMessage(data, origin); data:html5规范支持的任意基本类型或可复制的对象,但部分浏览器只支持字符串,所以传参时最好用JSON.stringify()序列化。origin:协议+主机+端口号,也可以设置为"*",表示可以传递给任意窗口,如果要指定和当前窗口同源的话设置为"/"。
getMessageHTML是我们对于要接受信息页面的引用,可以是iframe的contentWindow属性、window.open的返回值、通过name或下标从window.frames取到的值。
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros…
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><iframe id=ifr src=./child.html style="display: none;"></iframe><button id=btn>click</button><script>var btn = document.getElementById(btn);btn.addEventListener(click, function () {var ifr = document.getElementById(ifr);ifr.contentWindow.postMessage(0, *);}, false);</script></body></html>复制代码child.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title></head><body><script>window.addEventListener(message, function(event){alert(event.data);}, false);</script></body></html>复制代码点击index.html页面上的按钮,弹窗便会显示0。
六、跨域资源共享(CORS)
只要在服务端设置Access-Control-Allow-Origin就可以实现跨域请求,若是cookie请求,前后端都需要设置。由于同源策略的限制,所读取的cookie为跨域请求接口所在域的cookie,并非当前页的cookie。
CORS是目前主流的跨域解决方案。
原生node.js实现
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros… server.js命令启动本地服务器)
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title><scriptsrc=""integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo="crossorigin="anonymous"></script></head><body><script>$.get(:8080, function (data) {alert(data);});</script></body></html>复制代码server.js
var http = require(http);var server = http.createServer();server.on(request, function(req, res) {res.writeHead(200, {Access-Control-Allow-Credentials: true, // 后端允许发送CookieAccess-Control-Allow-Origin: https://mfaying.github.io,// 允许访问的域(协议+域名+端口)Set-Cookie: key=1;Path=/;Domain=mfaying.github.io;HttpOnly // HttpOnly:脚本无法读取cookie});res.write(JSON.stringify(req.method));res.end();});server.listen(8080);console.log(Server is running at port 8080...);复制代码koa结合koa2-cors中间件实现
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros… (访问前需要先执行node server.js命令启动本地服务器)
index.html
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Document</title><scriptsrc=""integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo="crossorigin="anonymous"></script></head><body><script>$.get(:8080, function (data) {alert(data);});</script></body></html>复制代码server.js
var koa = require(koa);var router = require(koa-router)();const cors = require(koa2-cors);var app = new koa();app.use(cors({origin: function (ctx) {if (ctx.url === /test) {return false;}return *;},exposeHeaders: [WWW-Authenticate, Server-Authorization],maxAge: 5,credentials: true,allowMethods: [GET, POST, DELETE],allowHeaders: [Content-Type, Authorization, Accept],}))router.get(/, async function (ctx) {ctx.body = "0";});app.use(router.routes()).use(router.allowedMethods());app.listen(3000);console.log(server is listening in port 3000);复制代码七、WebSocket协议跨域
WebSocket协议是HTML5的新协议。能够实现浏览器与服务器全双工通信,同时允许跨域,是服务端推送技术的一种很好的实现。
示例代码:github.com/mfaying/les… 演示网址:mfaying.github.io/lesson/cros… server.js命令启动本地websocket服务端)
前端代码:
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><meta http-equiv="X-UA-Compatible" content="ie=edge"><title>Document</title></head><body><script>var ws = new WebSocket(ws://localhost:8080/, echo-protocol);// 建立连接触发的事件ws.onopen = function () {var data = { "test": 1 };ws.send(JSON.stringify(data));// 可以给后台发送数据}; // 接收到消息的回调方法ws.onmessage = function (event) {alert(JSON.parse(event.data).test);} // 断开连接触发的事件ws.onclose = function () {conosle.log(close);};</script></body></html>复制代码server.js
var WebSocketServer = require(websocket).server;var http = require(http); var server = http.createServer(function(request, response) {response.writeHead(404);response.end();});server.listen(8080, function() {console.log(Server is listening on port 8080);}); wsServer = new WebSocketServer({httpServer: server,autoAcceptConnections: false}); function originIsAllowed(origin) {return true;} wsServer.on(request, function(request) {if (!originIsAllowed(request.origin)) {request.reject();console.log(Connection from origin + request.origin + rejected.);return;}var connection = request.accept(echo-protocol, request.origin);console.log(Connection accepted.);connection.on(message, function(message) {if (message.type === utf8) {const reqData = JSON.parse(message.utf8Data);reqData.test -= 1;connection.sendUTF(JSON.stringify(reqData));}});connection.on(close, function() {console.log(close);});});复制代码八、nginx代理跨域
原理:同源策略是浏览器的安全策略,不是HTTP协议的一部分。服务器端调用HTTP接口只是使用HTTP协议,不存在跨越问题。
实现:通过nginx配置代理服务器(域名与test1相同,端口不同)做跳板机,反向代理访问test2接口,且可以修改cookie中test信息,方便当前域cookie写入,实现跨域登录。
nginx具体配置:
#proxy服务器server {listen 81;server_namewww.test1.com;location / {proxy_pass :8080;#反向代理proxy_cookie_test www.test2.com www.test1.com; #修改cookie里域名indexindex.html index.htm;add_header Access-Control-Allow-Origin ;#当前端只跨域不带cookie时,可为*add_header Access-Control-Allow-Credentials true;}}复制代码转自: