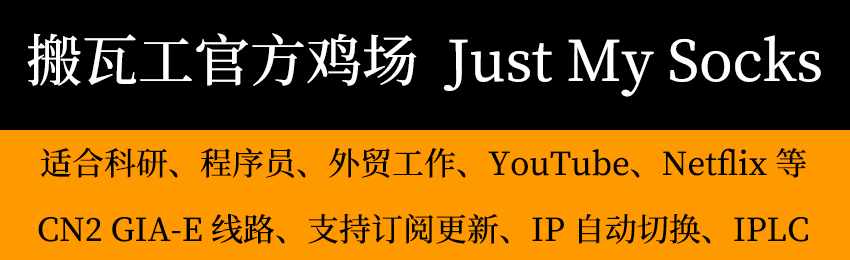
作者 | 朱卫军
Python有很多好用的函数和模块,这里给大家整理下我常用的一些方法及语句。
一、内置函数
内置函数是python自带的函数方法,拿来就可以用,比方说zip、filter、isinstance等。
下面是Python官档给出的内置函数列表,相当的齐全。
下面几个是常见的内置函数:
1、enumerate(iterable,start=0)
enumerate()是python的内置函数,是枚举、列举的意思
对于一个可迭代的(iterable)/可遍历的对象(如列表、字符串),enumerate将其组成一个索引序列,利用它可以同时获得索引和值
在python中enumerate的用法多用于在for循环中得到计数
seasons = [Spring, Summer, Fall, Winter]
list(enumerate(seasons))
[(0, Spring), (1, Summer), (2, Fall), (3, Winter)]
list(enumerate(seasons, start=1))
[(1, Spring), (2, Summer), (3, Fall), (4, Winter)]
2、zip(*iterables,strict=False)
zip() 函数用于将可迭代的对象作为参数,将对象中对应的元素打包成一个个元组,然后返回由这些元组组成的列表。
如果各个迭代器的元素个数不一致,则返回列表长度与最短的对象相同,利用 * 号操作符,可以将元组解压为列表。
zip(iterable1,iterable2, ...)
>>> for item in zip([1, 2, 3], [sugar, spice, everything nice]):... print(item)
...
(1, sugar)(2, spice)(3, everything nice)
3、filter(function,iterable)
filter是将一个序列进行过滤,返回迭代器的对象,去除不满足条件的序列。
filter(function,data)
function作为条件选择函数
比如说定义一个函数来检查输入数字是否为偶数。如果数字为偶数,它将返回True,否则返回False。
def is_even(x):if x % 2 == 0:return Trueelse:return False
然后使用filter对某个列表进行筛选:
l1 = [1, 2, 3, 4, 5]
fl = filter(is_even, l1)
list(fl)
4、isinstance(object,classinfo)
「isinstance」是用来判断某一个变量或者是对象是不是属于某种类型的一个函数
如果参数object是classinfo的实例,或者object是classinfo类的子类的一个实例, 返回True。如果object不是一个给定类型的的对象, 则返回结果总是False
>>>a = 2>>> isinstance (a,int)
True
>>> isinstance (a,str)
False
>>> isinstance (a,(str,int,list)) # 是元组中的一个返回 True
True
5、eval(expression[,globals[,locals]])
eval用来将字符串str当成有效的表达式来求值并返回计算结果
表达式解析参数expression并作为 Python 表达式进行求值(从技术上说是一个条件列表),采用globals和locals字典作为全局和局部命名空间。
>>>x = 7>>> eval( 3 * x )
21
>>> eval(pow(2,2))
4
>>> eval(2 + 2)
4
>>> n=81>>> eval("n + 4")
85
常用句式
在日常代码过程中,其实有很多常用的句式,出现频率非常高,也是大家约定俗成的写法。
「1、format字符串格式化」
format把字符串当成一个模板,通过传入的参数进行格式化,非常实用且强大
# 格式化字符串
print({} {}.format(hello,world))
# 浮点数
float1 = 563.78453
print("{:5.2f}".format(float1))
「2、连接字符串」
使用+连接两个字符串
string1 = "Linux"
string2 = "Hint"
joined_string = string1 + string2
print(joined_string)
「3、if...else条件语句」
Python 条件语句是通过一条或多条语句的执行结果(True 或者 False)来决定执行的代码块。
其中if...else语句用来执行需要判断的情形。
# Assign a numeric value
number = 70
# Check the is more than 70 or not
if (number >= 70):print("You have passed")else:print("You have not passed")
「4、for...in、while循环语句」
循环语句就是遍历一个序列,循环去执行某个操作,Python 中的循环语句有 for 和 while。
for循环
# Initialize the list
weekdays = ["Sunday", "Monday", "Tuesday","Wednesday", "Thursday","Friday", "Saturday"]print("Seven Weekdays are:\n")
# Iterate the list using for loop
for day in range(len(weekdays)):print(weekdays[day])
while循环
# Initialize counter
counter = 1
# Iterate the loop 5 times
while counter
# Print the counter value
print ("The current counter value: %d" % counter)
# Increment the counter
counter = counter + 1
「5、import导入其他脚本的功能」
有时需要使用另一个 python 文件中的脚本,这其实很简单,就像使用 import 关键字导入任何模块一样。
「vacations.py」
# Initialize values
vacation1 = "Summer Vacation"vacation2 = "Winter Vacation"
比如在下面脚本中去引用上面vacations.py中的代码
# Import another python script
import vacations as v
# Initialize the month list
months = ["January", "February", "March", "April", "May", "June","July", "August", "September", "October", "November", "December"]
# Initial flag variable to print summer vacation one time
flag = 0
# Iterate the list using for loop
for month in months:if month == "June" or month == "July":if flag == 0:print("Now",v.vacation1)
flag = 1
elif month == "December":print("Now",v.vacation2)else:print("The current month is",month)
「6、列表推导式」
Python 列表推导式是从一个或者多个迭代器快速简洁地创建数据类型的一种方法,它将循环和条件判断结合,从而避免语法冗长的代码,提高代码运行效率。能熟练使用推导式也可以间接说明你已经超越了 Python 初学者的水平。
# Create a list of characters using list comprehension
char_list = [ char for char in"linuxhint" ]print(char_list)
# Define a tuple of websites
websites = ("google.com","yahoo.com", "ask.com", "bing.com")
# Create a list from tuple using list comprehension
site_list = [ site for site in websites ]print(site_list)
「7、读写文件」
与计算的交互式Python最常使用的场景之一,比如去读取D盘中CSV文件,然后重新写入数据再保存。这就需要python执行读写文件的操作,这也是初学者要掌握的核心技能。
#Assign the filename
filename = "languages.txt"
# Open file for writing
fileHandler = open(filename, "w")
# Add some text
fileHandler.write("Bash\n")fileHandler.write("Python\n")fileHandler.write("PHP\n")
# Close the file
fileHandler.close()
# Open file for reading
fileHandler = open(filename, "r")
# Read a file line by line
for line in fileHandler:print(line)
# Close the file
fileHandler.close()
「8、切片和索引」
形如列表、字符串、元组等序列,都有切片和索引的需求,因为我们需要从中截取数据,所以这也是非常核心的技能。
var1 = Hello World!
var2 = "zhihu"print ("var1[0]: ", var1[0])print ("var2[1:5]: ", var2[1:5])
「9、使用函数和类」
函数和类是一种封装好的代码块,可以让代码更加简洁、实用、高效、强壮,是python的核心语法之一。
定义和调用函数
# Define addition function
def addition(number1, number2):
result = number1 + number2
print("Addition result:",result)
# Define area function with return statement
def area(radius):
result = 3.14 * radius * radius
return result
# Call addition function
addition(400, 300)
# Call area function
print("Area of the circle is",area(4))
定义和实例化类
# Define the class
class Employee:
name = "Mostak Mahmud"
# Define the method
def details(self):
print("Post: Marketing Officer")print("Department: Sales")print("Salary: $1000")
# Create the employee object
emp = Employee()
# Print the class variable
print("Name:",emp.name)
# Call the class method
emp.details()
「10、错误异常处理」
编程过程中难免会遇到错误和异常,所以我们要及时处理它,避免对后续代码造成影响。
所有的标准异常都使用类来实现,都是基类Exception的成员,都从基类Exception继承,而且都在exceptions模块中定义。
Python自动将所有异常名称放在内建命名空间中,所以程序不必导入exceptions模块即可使用异常。一旦引发而且没有捕捉SystemExit异常,程序执行就会终止。
异常的处理过程、如何引发或抛出异常及如何构建自己的异常类都是需要深入理解的。
# Try block
try:
# Take a number
number = int(input("Enter a number: "))if number % 2 == 0:print("Number is even")else:print("Number is odd")
# Exception block
except (ValueError):
# Print error message
print("Enter a numeric value")
小结
当然Python还有很多有用的函数和方法,需要大家自己去总结,这里抛砖引玉,希望能帮助到需要的小伙伴。